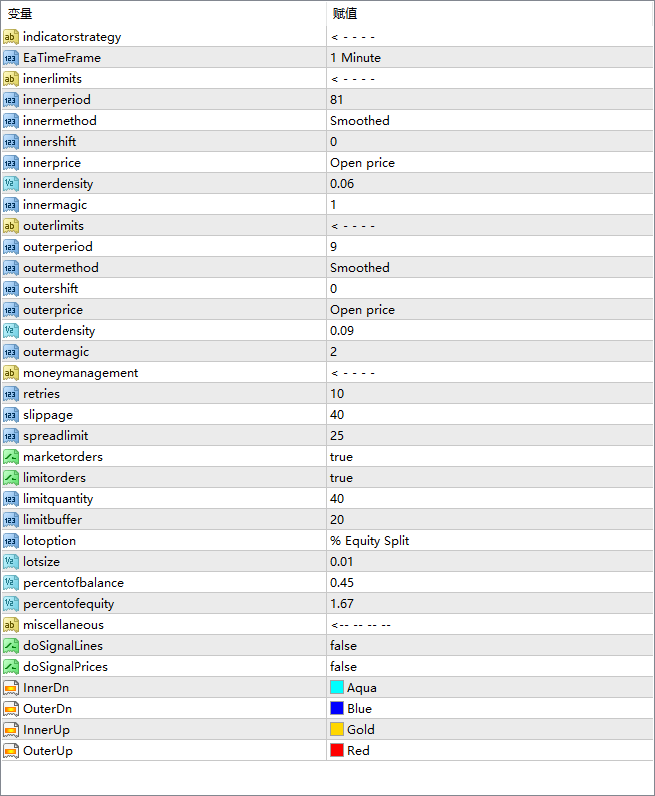
//+——————————————————————+
#property copyright “Copyright © 2018, Brian Lillard”
#property description “Density $calper”
#property link “https://www.mql5.com/en/users/subgenius/seller”
#property version “1.00”
#property strict
/*************************************************************||
*** GLOBAL VARIABLES *****************************************
**************************************************************/
//- indicator buffers
//- signal identifiers
const int SELL=2,
BUY=1,
NA=0;
//- new bar log
bool isNewBar;
int min,sec;
//-logs for performance
//- spread calculations
int intSpreadMultiplier;
//- signals
bool bOuterUpper,bOuterLower,bInnerUpper,bInnerLower;
bool SignalThisBar=false;
int Signal;
//- order info
bool OrdersOpen;
int iInnerB,iOuterB,iInnerS,iOuterS;
int iInnerBL,iOuterBL,iInnerSL,iOuterSL;
double CumulativePL;
string EaComment=”D$”;
/*************************************************************||
*** ENUMERATED LISTS *****************************************
**************************************************************/
enum OptLot{Fixed=0,/*Fixed Lot*/EquityFull=1,/*% Equity Full*/EquitySplit=2,/*% Equity Split*/BalanceFull=3,/*% Balance Full*/BalanceSplit=4,/*% Balance Split*/};
/*************************************************************||
*** EXTERNAL INPUTS ******************************************
**************************************************************/
input string indicatorstrategy = “< – – – – “;
input ENUM_TIMEFRAMES EaTimeFrame = PERIOD_M1;
bool trailexits = true;
input string innerlimits = “< – – – – “;
input int innerperiod = 81;
input ENUM_MA_METHOD innermethod = MODE_SMMA;
input int innershift = 0;
input ENUM_APPLIED_PRICE innerprice = PRICE_OPEN;
input double innerdensity = 0.06;
input int innermagic = 1;
input string outerlimits = “< – – – – “;
input int outerperiod = 9;
input ENUM_MA_METHOD outermethod = MODE_SMMA;
input int outershift = 0;
input ENUM_APPLIED_PRICE outerprice = PRICE_OPEN;
input double outerdensity = 0.09;
input int outermagic = 2;
input string moneymanagement = “< – – – – “;
input int retries = 10;
input int slippage = 40;
input int spreadlimit = 25;
input bool marketorders = TRUE;
input bool limitorders = TRUE;
input int limitquantity = 40;
input int limitbuffer = 20;
input OptLot lotoption = EquitySplit;
input double lotsize = 0.01;
input double percentofbalance = 0.45;
input double percentofequity = 1.67;
input string miscellaneous = “<– — — — “;
bool doLabels = FALSE;
color FontColor = DarkKhaki;
string Font = “Calibri”;
int FontSize = 7;
int Corner = 1;
input bool doSignalLines = FALSE;
input bool doSignalPrices = FALSE;
input color InnerDn = Aqua;
input color OuterDn = Blue;
input color InnerUp = Gold;
input color OuterUp = Red;
/*************************************************************||
*** INDICATOR CODE *******************************************
**************************************************************/
double inner(int buffer,int bar){ return( iEnvelopes(NULL,0,innerperiod,innermethod,innershift,innerprice,innerdensity,buffer,bar) ); }
double outer(int buffer,int bar){ return( iEnvelopes(NULL,0,outerperiod,outermethod,outershift,outerprice,outerdensity,buffer,bar) ); }
void getSignals(int i)
{
Signal=NA;
double OuterUpper=outer(MODE_UPPER,i); bOuterUpper=false;
double OuterLower=outer(MODE_LOWER,i); bOuterLower=false;
double InnerUpper=inner(MODE_UPPER,i); bInnerUpper=false;
double InnerLower=inner(MODE_LOWER,i); bInnerLower=false;
if( Low[i]<=OuterLower && High[i]>=OuterLower ) //outer signals
{ Signal=BUY; bOuterLower=TRUE;
if(doSignalLines){ VLINE(“VLINEO”+IntegerToString(i),Time[i],Red,3); }
if(doSignalPrices){ ARROW(“ARROWO”+IntegerToString(i),Time[i],OuterLower,4,OuterUp); } }
if( High[i]>=OuterUpper && Low[i]<=OuterUpper )
{ Signal=SELL; bOuterUpper=TRUE;
if(doSignalLines){ VLINE(“VLINEO”+IntegerToString(i),Time[i],Blue,3); }
if(doSignalPrices){ ARROW(“ARROWO”+IntegerToString(i),Time[i],OuterUpper,4,OuterDn); } }
if( OuterUpper>InnerUpper && OuterLower<InnerLower ) //inner signals
{
if( Low[i]<=InnerLower && High[i]>=InnerLower )
{ Signal=BUY; bInnerLower=TRUE;
if(doSignalLines){ VLINE(“VLINEI”+IntegerToString(i),Time[i],Gold,1); }
if(doSignalPrices){ ARROW(“ARROWI”+IntegerToString(i),Time[i],InnerLower,4,InnerUp); } }
if( High[i]>=InnerUpper && Low[i]<=InnerUpper )
{ Signal=SELL; bInnerUpper=TRUE;
if(doSignalLines){ VLINE(“VLINEI”+IntegerToString(i),Time[i],Aqua,1); }
if(doSignalPrices){ ARROW(“ARROWI”+IntegerToString(i),Time[i],InnerUpper,4,InnerDn); } }
}
}
/*************************************************************||
*** MINI-FUNCTIONS *******************************************
**************************************************************/
bool bGoodSpread(){ if((spreadlimit>0 && NormalizeDouble((Ask-Bid)*intSpreadMultiplier,1)<=spreadlimit) || spreadlimit<=0){return(TRUE);} return(FALSE); }
bool bWrongTF(){ if(EaTimeFrame!=0){ if(strPeriod(Period())!=strPeriod(EaTimeFrame)){ return(TRUE); } } return(false); }
string strSignal(int iSS){if(iSS==1 || iSS==3){return(“BUY”);}if(iSS==2 || iSS==4){return(“SELL”);}return(“NA”);}
bool bNewBar(){ static datetime B1T; if(B1T!=Time[0]){ B1T=Time[0]; return(true); } return(false); }
bool Expire(){ if(CurTime()>=StrToTime(“2018.08.11”)){return(TRUE);} return(false); }
string strPeriod(int Per)
{
if(Per==0){ Per=Period(); } if(Per==1){ return(“M1”); } if(Per==5){ return(“M5”); }
if(Per==15){ return(“M15”); } if(Per==30){ return(“M30”); } if(Per==60){ return(“H1”); }
if(Per==240){ return(“H4”); } if(Per==1440){ return(“D1”); } if(Per==10080){ return(“W1”); }
if(Per==43200){ return(“MN”); } return(“{ Unknown Timeframe }”);
}
/*************************************************************||
*** OPEN AND CLOSED TRADE POOL *******************************
**************************************************************/
bool OpenOrdersFound()
{
iInnerBL=0; iOuterBL=0; iInnerSL=0; iOuterSL=0;
iInnerB=0; iOuterB=0; iInnerS=0; iOuterS=0;
CumulativePL=0;
int OrdersOT=OrdersTotal();
for(int X=0; X<OrdersOT; X++)
{
if(!OrderSelect(X,SELECT_BY_POS,MODE_TRADES) ){continue;}
if( OrderMagicNumber()==innermagic )
{
if( OrderType()==OP_SELLLIMIT ){ iInnerSL++; }else if( OrderType()==OP_BUYLIMIT ){ iInnerBL++; }
if( OrderType()==OP_SELL ){ iInnerS++; }else if( OrderType()==OP_BUY ){ iInnerB++; }
}
else
{
if( OrderMagicNumber()==outermagic )
{
if( OrderType()==OP_SELLLIMIT ){ iOuterSL++; }else if( OrderType()==OP_BUYLIMIT ){ iOuterBL++; }
if( OrderType()==OP_SELL ){ iOuterS++; }else if( OrderType()==OP_BUY ){ iOuterB++; }
}
}
CumulativePL+=OrderProfit()+OrderCommission()+OrderSwap();
}
if( iInnerS+iInnerB+iOuterS+iOuterB>0 ){ return(true); }
return(false);
}
/*************************************************************||
*** INITIALIZE ***********************************************
**************************************************************/
int OnInit()
{
if( Expire() ){ return(0); }
else{ Print(“Trial version expires 2018.08.11 – Copyright© 2018, Brian Lillard( [email protected] )”); }
if( doSignalLines || doSignalPrices ){ for(int i=Bars-1; i>=0; i–){ getSignals(i); } }
switch(Digits)
{
case 5: intSpreadMultiplier=100000; break;
case 4: intSpreadMultiplier= 10000; break;
case 3: intSpreadMultiplier= 1000; break;
case 2: intSpreadMultiplier= 100; break;
case 1: intSpreadMultiplier= 10; break;
default: break;
}
return(INIT_SUCCEEDED);
}
/*************************************************************||
*** ONTICK ***************************************************
**************************************************************/
void OnTick()
{
if( Expire() ){ Comment(“The trial version has expired!”); return; }
if(bWrongTF()){ Comment(“ERROR: Wrong Timeframe”); return; }
isNewBar=bNewBar(); if(isNewBar){ if( CumulativePL>0 ){ CloseAllOrders(innermagic); CloseAllOrders(outermagic); } SignalThisBar=false; }
OrdersOpen=OpenOrdersFound();
getSignals(0);
if( CumulativePL>0 ){ CloseAllOrders(innermagic); CloseAllOrders(outermagic); }
//if alternate inner signal then delete all inner signal expiry, close, and open new positions
//if alternate outer signal then delete all outer signal expiry, close, and open new positions
if( Signal!=0 && !SignalThisBar && bGoodSpread() )
{
switch(Signal)
{
case 1: //BUY
if( bInnerLower )
{
if( iInnerSL+iOuterSL>0 ){ DeleteOrders(innermagic); }
if( iInnerS+iOuterS>0 ){ CloseAllOrders(innermagic); }
Trade(innermagic);
}
if( bOuterLower )
{
if( iInnerSL+iOuterSL>0 ){ DeleteOrders(innermagic); }
if( iInnerS+iOuterS>0 ){ CloseAllOrders(innermagic); }
Trade(outermagic);
}
break;
case 2: //SELL
if( bInnerUpper )
{
if( iInnerBL+iOuterBL>0 ){ DeleteOrders(outermagic); }
if( iInnerB+iOuterB>0 ){ CloseAllOrders(outermagic); }
Trade(innermagic);
}
if( bOuterUpper )
{
if( iInnerBL+iOuterBL>0 ){ DeleteOrders(outermagic); }
if( iInnerB+iOuterB>0 ){ CloseAllOrders(outermagic); }
Trade(outermagic);
}
break;
default: break;
}
}
}
/*************************************************************||
*** DEINITIALIZE *********************************************
**************************************************************/
void OnDeinit(const int reason)
{
if( doSignalLines )
{
for(int i=Bars; i>=0; i–)
{
ObjectDelete(0,”VLINEO”+IntegerToString(i));
ObjectDelete(0,”VLINEI”+IntegerToString(i));
ObjectDelete(0,”ARROWO”+IntegerToString(i));
ObjectDelete(0,”ARROWI”+IntegerToString(i));
}
}
HideTestIndicators(true);
Comment(“”);
}
/*************************************************************||
*** TRAIL POSITIONS ******************************************
**************************************************************/
/*************************************************************||
*** MANAGE EXPIRY ********************************************
**************************************************************/
void DeleteOrders(int iMagic)
{
for(int OrdersOT=OrdersTotal()-1; OrdersOT>=0; OrdersOT–)
{
if(!OrderSelect(OrdersOT,SELECT_BY_POS,MODE_TRADES) || OrderMagicNumber()!=iMagic || OrderType()<2){continue;}
bool del=OrderDelete(OrderTicket()); ObjectsDeleteAll(0,0,OBJ_ARROW);
if(!del){ Print(“Deletion of Expired Order error: “,strError(GetLastError())); }
}
}
/*************************************************************||
*** EXIT POSITIONS *******************************************
**************************************************************/
void CloseAllOrders(int iMagic)
{
bool result=false; int iRetries;
for(int OrdersOT=OrdersTotal()-1; OrdersOT>=0; OrdersOT–)
{
if(!OrderSelect(OrdersOT,SELECT_BY_POS,MODE_TRADES) || OrderMagicNumber()!=iMagic || OrderType()>1){continue;}
result=false; iRetries=1;
while( iRetries<=retries && !result)
{
while(IsTradeContextBusy()){Sleep(10);} result=OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),slippage,clrNONE); iRetries++;
}
if(!result){Print(“Failed To Close Order#”+IntegerToString(OrderTicket())+”. Error: “+strError(GetLastError())+”.” ); }
}
}
/*************************************************************||
*** OPEN POSITIONS *******************************************
**************************************************************/
void Trade(int iMagic)
{
if(IsStopped() || !IsTradeAllowed()){ return; }
while(IsTradeContextBusy()){ Sleep(10); }
string sEAC=EaComment+”,”+strPeriod(Period())+”,”+DoubleToStr((Ask-Bid)*intSpreadMultiplier,1);
double SL=0,TP=0; double OpenPrice;
int ticket=0,iRetries=1;
switch(Signal)
{
case 1: //BUY
while( marketorders && ticket<=0 && iRetries<=retries )
{
ticket=OrderSend(Symbol(),OP_BUY,dNextLot(),Ask,slippage,0,0,sEAC,iMagic,0,MediumOrchid); iRetries++;
} if( marketorders && ticket<=0 ){ Print(“OrderSend Error: “,strError(GetLastError())); }
if( limitorders )
{
if( innerprice==PRICE_OPEN && outerprice==PRICE_OPEN ){ OpenPrice=Open[0]; }else{ OpenPrice=Ask; }
for( int i=1; i<=limitquantity; i++ )
{
ticket=OrderSend(Symbol(),OP_BUYLIMIT,dNextLot(),OpenPrice-(limitbuffer*Point()*(i)),slippage,0,0,sEAC,iMagic,0,MediumOrchid);
}
}
break;
case 2: //SELL
while( marketorders && ticket<=0 && iRetries<=retries )
{
ticket=OrderSend(Symbol(),OP_SELL,dNextLot(),Bid,slippage,0,0,sEAC,iMagic,0,Orange); iRetries++;
} if( marketorders && ticket<=0 ){ Print(“OrderSend Error: “,strError(GetLastError())); }
if( limitorders )
{
if( innerprice==PRICE_OPEN && outerprice==PRICE_OPEN ){ OpenPrice=Open[0]; }else{ OpenPrice=Bid; }
for( int i=1; i<=limitquantity; i++ )
{
ticket=OrderSend(Symbol(),OP_SELLLIMIT,dNextLot(),OpenPrice+(limitbuffer*Point()*(i)),slippage,0,0,sEAC,iMagic,0,Orange);
}
}
break;
default: break;
}
/*
if(ticket>0 && (TP>0 || SL>0))
{
while(IsTradeContextBusy()){Sleep(10);}
for(iRetries=retries; iRetries>0; iRetries–){ if(OrderModify(ticket,OrderOpenPrice(),SL,TP,0,clrNONE)){return;} }
}
*/
}
/*************************************************************||
*** MONEY MANAGEMENT *****************************************
**************************************************************/
double dNextLot()
{
int market=0; if(marketorders){ market=1; }
double Lot=0;
//rountine lots
if( lotoption==BalanceFull || lotoption==BalanceSplit ){ Lot=percentofbalance*AccountBalance()/10000; }
if( lotoption==EquityFull || lotoption==EquitySplit ){ Lot = percentofequity*AccountEquity()/10000; }
if(marketorders && limitorders && limitquantity>=1 && (lotoption==EquitySplit || lotoption==BalanceSplit)){ Lot=Lot/(limitquantity+market); }
//normalize bulk lot
if( Lot<NormalizeDouble(MarketInfo(Symbol(),MODE_MINLOT),2) ){ Lot=NormalizeDouble(MarketInfo(Symbol(),MODE_MINLOT),2); }
if( Lot>NormalizeDouble(MarketInfo(Symbol(),MODE_MAXLOT),2) ){ Lot=NormalizeDouble(MarketInfo(Symbol(),MODE_MAXLOT),2); }
return(NormalizeDouble(Lot,2));
}
/*************************************************************||
*** LABEL CODE ***********************************************
**************************************************************/
void ManageLabels()
{
//LABEL(“LABEL1”,”Spread/Max :: “+DoubleToString(MarketInfo(Symbol(),MODE_SPREAD),0)+”/”+DoubleToString(spreadlimit,1),9,18);
//LABEL(“LABEL2″,”Profit/Loss :: ” );
//+DoubleToString(CumulativePL,2),9,28);
//LABEL(“LABEL3″,”Sell Trades :: ” );
//+IntegerToString(intSellsOpen),9,38);
//LABEL(“LABEL4″,”Buy Trades :: ” );
//+IntegerToString(intBuysOpen),9,48);
//LABEL(“LABEL5″,”Trend :: ” );
//+strSignal(TMACMACD(0)),9,58);
//LABEL(“LABEL6″,”Signal :: ” );
//+strSignal(OHLCBREAKZ(0)),9,68);
}
void LABEL(string sName, string sText, int iX, int iY)
{
if(ObjectFind(sName)==-1){ ObjectCreate(sName,OBJ_LABEL,0,0,0); }
ObjectSetText(sName,sText,FontSize,Font,FontColor); ObjectSetString(0,sName,OBJPROP_TOOLTIP,sText);
ObjectSet(sName,OBJPROP_SELECTABLE,0); ObjectSet(sName,OBJPROP_CORNER,Corner);
ObjectSet(sName,OBJPROP_XDISTANCE,iX); ObjectSet(sName,OBJPROP_YDISTANCE,iY);
}
/*************************************************************||
*** ERROR MESSAGES *******************************************
**************************************************************/
string strError(int iError)
{
switch(iError)
{
case 0: return(“0: No Error”);
case 1: return(“1: No Error, Trade Conditions Not Changed”);
case 2: return(“2: Common Error”);
case 3: return(“3: Invalid Trade Parameters”);
case 4: return(“4: Trade Server Is Busy”);
case 5: return(“5: Old Version Of The Client Terminal”);
case 6: return(“6: No Connection With Trade Server”);
case 7: return(“7: Not Enough Rights”);
case 8: return(“8: Too Frequent Requests”);
case 9: return(“9: Malfunctional Rrade Operation(Never Returned Error)”);
case 64: return(“64: Account Disabled”);
case 65: return(“65: Invalid Account”);
case 128: return(“128: Trade Timeout”);
case 129: return(“129: Invalid Price”);
case 130: return(“130: Invalid Stops”);
case 131: return(“131: Invalid Trade Volume”);
case 132: return(“132: Market Is Closed”);
case 133: return(“133: Trade Is Disabled”);
case 134: return(“134: Not Enough Money”);
case 135: return(“135: Price Changed”);
case 136: return(“136: Off Quotes”);
case 137: return(“137: Broker Is Busy(Never Returned Error)”);
case 138: return(“138: Requote”);
case 139: return(“139: Order Is Locked”);
case 140: return(“140: Long Positions Only Allowed”);
case 141: return(“141: Too Many Requests”);
case 145: return(“145 Modification Denied Because Order Is Too Close To Market”);
case 146: return(“146: Trade Context Is Busy”);
case 147: return(“147: Expirations Are Denied By Broker”);
case 148: return(“148: Amount Of Open And Pending Orders Has Reached The Limit”);
case 149: return(“149: Hedging Is Prohibited”);
case 150: return(“150: Prohibited By FIFO Rules”);
}
return(“Unknown Error”);
}
void VLINE(string sName,datetime dTime,color cColor,int iWidth)
{
if(ObjectFind(sName)==-1){ ObjectCreate(sName,OBJ_VLINE,0,dTime,0,0); }
ObjectSet(sName,OBJPROP_COLOR,cColor);
ObjectSet(sName,OBJPROP_WIDTH,iWidth);
ObjectSet(sName,OBJPROP_BACK,true);
}
void ARROW(string sName,datetime dTime,double dPrice,int iArrow,color cColor)
{
if(ObjectFind(sName)==-1){ ObjectCreate(sName,OBJ_ARROW,0,0,0); }
ObjectSet(sName,OBJPROP_ARROWCODE,iArrow);
ObjectSet(sName,OBJPROP_TIME1,dTime);
ObjectSet(sName,OBJPROP_PRICE1,dPrice);
ObjectSet(sName,OBJPROP_COLOR,cColor);
if( cColor==InnerUp ){ ObjectSetString(0,sName,OBJPROP_TOOLTIP,”Inner Limit Buy”); }
if( cColor==InnerDn ){ ObjectSetString(0,sName,OBJPROP_TOOLTIP,”Inner Limit Sell”); }
if( cColor==OuterUp ){ ObjectSetString(0,sName,OBJPROP_TOOLTIP,”Outer Limit Buy”); }
if( cColor==OuterDn ){ ObjectSetString(0,sName,OBJPROP_TOOLTIP,”Outer Limit Sell”); }
}
DensityScalperEA这个源码下载地址打不开了
下载链接已经更新